LPC1768: Lcd 4bit
In this tutorial we are going to see how to interface a 2x16 LCD with LPC1768 in 4-bit mode. As per the name the 2x16 has 2 lines with 16 chars on each lines. It supports all the ascii chars and is basically used for displaying the alpha numeric characters. Here each character is displayed in a matrix of 5x7 pixels. Apart from alpha numeric chars it also provides the provision to display the custom characters by creating the pattern. Scope of this tutorial is to show how to display the alpha numeric chars on LCD, Generating and displaying the custom chars will be discussed in subsequent tutorials.
Contents
[hide]LCD UNIT
Let us look at a pin diagram of a commercially available LCD like JHD162 which uses a HD44780 controller and then describe its operation.
Pin Number | Symbol | Pin Function |
1 | VSS | Ground |
2 | VCC | +5v |
3 | VEE | Contrast adjustment (VO) |
4 | RS | Register Select. 0:Command, 1: Data |
5 | R/W | Read/Write, R/W=0: Write & R/W=1: Read |
6 | EN | Enable. Falling edge triggered |
7 | D0 | Data Bit 0 (Not used in 4-bit operation) |
8 | D1 | Data Bit 1 (Not used in 4-bit operation) |
9 | D2 | Data Bit 2 (Not used in 4-bit operation) |
10 | D3 | Data Bit 3 (Not used in 4-bit operation) |
11 | D4 | Data Bit 4 |
12 | D5 | Data Bit 5 |
13 | D6 | Data Bit 6 |
14 | D7 | Data Bit 7/Busy Flag |
15 | A/LED+ | Back-light Anode(+) |
16 | K/LED- | Back-Light Cathode(-) |
Apart from the voltage supply connections the important pins from the programming perspective are the data lines(8-bit Data bus), Register select, Read/Write and Enable pin.
Data Bus: As shown in the above figure and table, an alpha numeric lcd has a 8-bit data bus referenced as D0-D7.
As it is a 8-bit data bus, we can send the data/cmd to LCD in bytes. It also provides the provision to send the the data/cmd in chunks of 4-bit, which is used when there are limited number of GPIO lines on the microcontroller.
Register Select(RS): The LCD has two register namely a Data register and Command register. Any data that needs to be displayed on the LCD has to be written to the data register of LCD. Command can be issued to LCD by writing it to Command register of LCD.
This signal is used to differentiate the data/cmd received by the LCD.
If the RS signal is LOW then the LCD interprets the 8-bit info as Command and writes it Command register and performs the action as per the command.
If the RS signal is HIGH then the LCD interprets the 8-bit info as data and copies it to data register. After that the LCD decodes the data for generating the 5x7 pattern and finally displays on the LCD.
Read/Write(RW): This signal is used to write the data/cmd to LCD and reads the busy flag of LCD.
For write operation the RW should be LOW and for read operation the R/W should be HIGH.
Enable(EN): This pin is used to send the enable trigger to LCD.
After sending the data/cmd, Selecting the data/cmd register, Selecting the Write operation. A HIGH-to-LOW pulse has to be send on this enable pin which will latch the info into the LCD register and triggers the LCD to act accordingly.
Hardware Connections
Schematic
Below schematic shows the minimum connection required for interfacing the LCD with the microcontroller.
As we are interfacing the LCD in 4-bit mode, only the higher 4 data lines are used as data bus.
Port Connection
This section shows how to configure the GPIO for interfacing the LCD.
The below configuration is as per the above schematic. You can connect the LCD to any of the PORT pins available on your boards and update this section accordingly
/* Configure the data bus and Control bus as per the hardware connection */ | |
#define LcdDataBusPort LPC_GPIO1->FIOPIN | |
#define LcdControlBusPort LPC_GPIO2->FIOPIN | |
#define LcdDataBusDirnReg LPC_GPIO1->FIODIR | |
#define LcdCtrlBusDirnReg LPC_GPIO2->FIODIR | |
#define LCD_D4 24 | |
#define LCD_D5 25 | |
#define LCD_D6 26 | |
#define LCD_D7 27 | |
#define LCD_RS 0 | |
#define LCD_RW 1 | |
#define LCD_EN 2 |
LCD Operation
In this section we are going to see how to send the data/cmd to the LCD along with the timing diagrams. First lets see the timing diagram for sending the data and the command signals(RS,RW,EN), accordingly we write the algorithm and finally the code.
Timing Diagram
The below image shows the timing diagram for sending the data to the LCD.
As shown in the timing diagram the data is written after sending the RS and RW signals. It is still ok to send the data before these signals.
The only important thing is the data should be available on the databus before generating the High-to-Low pulse on EN pin.
Steps for Sending Command:
- Send the I/P command to LCD.
- Select the Control Register by making RS low.
- Select Write operation making RW low.
- Send a High-to-Low pulse on Enable PIN with some delay_us.
/* Function to send the command to LCD. As it is 4bit mode, a byte of data is sent in two 4-bit nibbles */ | |
void Lcd_CmdWrite(char cmd) | |
{ | |
sendNibble((cmd >> 0x04) & 0x0F); //Send higher nibble | |
LcdControlBusPort &= ~(1<<LCD_RS); // Send LOW pulse on RS pin for selecting Command register | |
LcdControlBusPort &= ~(1<<LCD_RW); // Send LOW pulse on RW pin for Write operation | |
LcdControlBusPort |= (1<<LCD_EN); // Generate a High-to-low pulse on EN pin | |
delay(1000); | |
LcdControlBusPort &= ~(1<<LCD_EN); | |
delay(10000); | |
sendNibble(cmd & 0x0F); //Send Lower nibble | |
LcdControlBusPort &= ~(1<<LCD_RS); // Send LOW pulse on RS pin for selecting Command register | |
LcdControlBusPort &= ~(1<<LCD_RW); // Send LOW pulse on RW pin for Write operation | |
LcdControlBusPort |= (1<<LCD_EN); // Generate a High-to-low pulse on EN pin | |
delay(1000); | |
LcdControlBusPort &= ~(1<<LCD_EN); | |
delay(10000); | |
} |
Steps for Sending Data:
- Send the character to LCD.
- Select the Data Register by making RS high.
- Select Write operation making RW low.
- Send a High-to-Low pulse on Enable PIN with some delay_us.
The timings are similar as above only change is that RS is made high for selecting Data register.
/* Function to send the data to LCD. As it is 4bit mode, a byte of data is sent in two 4-bit nibbles */ | |
void Lcd_DataWrite(char dat) | |
{ | |
sendNibble((dat >> 0x04) & 0x0F); //Send higher nibble | |
LcdControlBusPort |= (1<<LCD_RS); // Send HIGH pulse on RS pin for selecting data register | |
LcdControlBusPort &= ~(1<<LCD_RW); // Send LOW pulse on RW pin for Write operation | |
LcdControlBusPort |= (1<<LCD_EN); // Generate a High-to-low pulse on EN pin | |
delay(1000); | |
LcdControlBusPort &= ~(1<<LCD_EN); | |
delay(10000); | |
sendNibble(dat & 0x0F); //Send higher nibble | |
LcdControlBusPort |= (1<<LCD_RS); // Send HIGH pulse on RS pin for selecting data register | |
LcdControlBusPort &= ~(1<<LCD_RW); // Send LOW pulse on RW pin for Write operation | |
LcdControlBusPort |= (1<<LCD_EN); // Generate a High-to-low pulse on EN pin | |
delay(1000); | |
LcdControlBusPort &= ~(1<<LCD_EN); | |
delay(10000); | |
} |
Code Examples
Example 1
Here is the complete code for displaying the data on 2x16 LCD in 4-bit mode.
#include<lpc17xx.h> | |
/* Configure the data bus and Control bus as per the hardware connection */ | |
#define LcdDataBusPort LPC_GPIO1->FIOPIN | |
#define LcdControlBusPort LPC_GPIO2->FIOPIN | |
#define LcdDataBusDirnReg LPC_GPIO1->FIODIR | |
#define LcdCtrlBusDirnReg LPC_GPIO2->FIODIR | |
#define LCD_D4 24 | |
#define LCD_D5 25 | |
#define LCD_D6 26 | |
#define LCD_D7 27 | |
#define LCD_RS 0 | |
#define LCD_RW 1 | |
#define LCD_EN 2 | |
/* Masks for configuring the DataBus and Control Bus direction */ | |
#define LCD_ctrlBusMask ((1<<LCD_RS)|(1<<LCD_RW)|(1<<LCD_EN)) | |
#define LCD_dataBusMask ((1<<LCD_D4)|(1<<LCD_D5)|(1<<LCD_D6)|(1<<LCD_D7)) | |
/* local function to generate some delay */ | |
void delay(int cnt) | |
{ | |
int i; | |
for(i=0;i<cnt;i++); | |
} | |
/* Function send the a nibble on the Data bus (LCD_D4 to LCD_D7) */ | |
void sendNibble(char nibble) | |
{ | |
LcdDataBusPort&=~(LCD_dataBusMask); // Clear previous data | |
LcdDataBusPort|= (((nibble >>0x00) & 0x01) << LCD_D4); | |
LcdDataBusPort|= (((nibble >>0x01) & 0x01) << LCD_D5); | |
LcdDataBusPort|= (((nibble >>0x02) & 0x01) << LCD_D6); | |
LcdDataBusPort|= (((nibble >>0x03) & 0x01) << LCD_D7); | |
} | |
/* Function to send the command to LCD. | |
As it is 4bit mode, a byte of data is sent in two 4-bit nibbles */ | |
void Lcd_CmdWrite(char cmd) | |
{ | |
sendNibble((cmd >> 0x04) & 0x0F); //Send higher nibble | |
LcdControlBusPort &= ~(1<<LCD_RS); // Send LOW pulse on RS pin for selecting Command register | |
LcdControlBusPort &= ~(1<<LCD_RW); // Send LOW pulse on RW pin for Write operation | |
LcdControlBusPort |= (1<<LCD_EN); // Generate a High-to-low pulse on EN pin | |
delay(1000); | |
LcdControlBusPort &= ~(1<<LCD_EN); | |
delay(10000); | |
sendNibble(cmd & 0x0F); //Send Lower nibble | |
LcdControlBusPort &= ~(1<<LCD_RS); // Send LOW pulse on RS pin for selecting Command register | |
LcdControlBusPort &= ~(1<<LCD_RW); // Send LOW pulse on RW pin for Write operation | |
LcdControlBusPort |= (1<<LCD_EN); // Generate a High-to-low pulse on EN pin | |
delay(1000); | |
LcdControlBusPort &= ~(1<<LCD_EN); | |
delay(10000); | |
} | |
void Lcd_DataWrite(char dat) | |
{ | |
sendNibble((dat >> 0x04) & 0x0F); //Send higher nibble | |
LcdControlBusPort |= (1<<LCD_RS); // Send HIGH pulse on RS pin for selecting data register | |
LcdControlBusPort &= ~(1<<LCD_RW); // Send LOW pulse on RW pin for Write operation | |
LcdControlBusPort |= (1<<LCD_EN); // Generate a High-to-low pulse on EN pin | |
delay(1000); | |
LcdControlBusPort &= ~(1<<LCD_EN); | |
delay(10000); | |
sendNibble(dat & 0x0F); //Send Lower nibble | |
LcdControlBusPort |= (1<<LCD_RS); // Send HIGH pulse on RS pin for selecting data register | |
LcdControlBusPort &= ~(1<<LCD_RW); // Send LOW pulse on RW pin for Write operation | |
LcdControlBusPort |= (1<<LCD_EN); // Generate a High-to-low pulse on EN pin | |
delay(1000); | |
LcdControlBusPort &= ~(1<<LCD_EN); | |
delay(10000); | |
} | |
int main() | |
{ | |
char i,a[]={"Good morning!"}; | |
SystemInit(); //Clock and PLL configuration | |
LcdDataBusDirnReg |= LCD_dataBusMask; // Configure all the LCD pins as output | |
LcdCtrlBusDirnReg |= LCD_ctrlBusMask; | |
Lcd_CmdWrite(0x02); // Initialize Lcd in 4-bit mode | |
Lcd_CmdWrite(0x28); // enable 5x7 mode for chars | |
Lcd_CmdWrite(0x0E); // Display OFF, Cursor ON | |
Lcd_CmdWrite(0x01); // Clear Display | |
Lcd_CmdWrite(0x80); // Move the cursor to beginning of first line | |
Lcd_DataWrite('H'); | |
Lcd_DataWrite('e'); | |
Lcd_DataWrite('l'); | |
Lcd_DataWrite('l'); | |
Lcd_DataWrite('o'); | |
Lcd_DataWrite(' '); | |
Lcd_DataWrite('w'); | |
Lcd_DataWrite('o'); | |
Lcd_DataWrite('r'); | |
Lcd_DataWrite('l'); | |
Lcd_DataWrite('d'); | |
Lcd_CmdWrite(0xc0); | |
for(i=0;a[i]!=0;i++) | |
{ | |
Lcd_DataWrite(a[i]); | |
} | |
while(1); | |
} |
Using Explore Embedded Libraries :
In the above tutorial we just discussed how to interface 2x16Lcd in 4-bit mode.
Once you know the working of lcd, you can directly use the ExploreEmbedded libraries to play around with your LCD.
For that you need to include the lcd.c/lcd.h and the associated files(delay/stdutils).
After including these files, the only thing you got to do is to configure the PORTs in lcd.h as per your hardware connection.
The below sample code shows how to use the already available LCD functions.
Refer this link for more info on LCD libraries.
#include<lpc17xx.h> | |
#include "lcd.h" //User defined LCD library which contains the lcd routines | |
#include "delay.h" //User defined library which contains the delay routines | |
void main() | |
{ | |
SystemInit(); //Clock and PLL configuration | |
/* Setup/Map the controller pins for LCD operation, In 4bit mode D0-D3 are P_NC(Not Connected) | |
RS RW EN D0 D1 D2 D3 D4 D5 D6 D7*/ | |
LCD_SetUp(P2_0,P2_1,P2_2,P_NC,P_NC,P_NC,P_NC,P1_24,P1_25,P1_26,P1_27); | |
/* Specify the LCD type(2x16, 4x16 etc) for initialization*/ | |
LCD_Init(2,16); | |
while(1) | |
{ | |
/*scroll the message on line1 */ | |
LCD_Clear(); | |
LCD_ScrollMessage(1," Program to demonstrate the ExploreEmbedded Lib Usage"); | |
LCD_Clear(); | |
LCD_Printf("Hello, World \nGood Morning"); | |
DELAY_sec(3); | |
LCD_Clear(); | |
LCD_Printf("DecNum:%4d",1234); | |
LCD_Printf("\nHexNum:%4x",0xABCD); | |
DELAY_sec(3); | |
LCD_Clear(); | |
LCD_Printf("16-bit Bin:1234"); | |
LCD_GoToNextLine(); | |
LCD_Printf(" %16b",0x1234); | |
DELAY_sec(3); | |
} | |
} |
Downloads
Download the complete project folder from the below link:
https://codeload.github.com/ExploreEmbedded/Explore-Cortex-M3-LPC1768-Stick-DVB-14001/zip/master
Have a opinion, suggestion , question or feedback about the article let it out here!
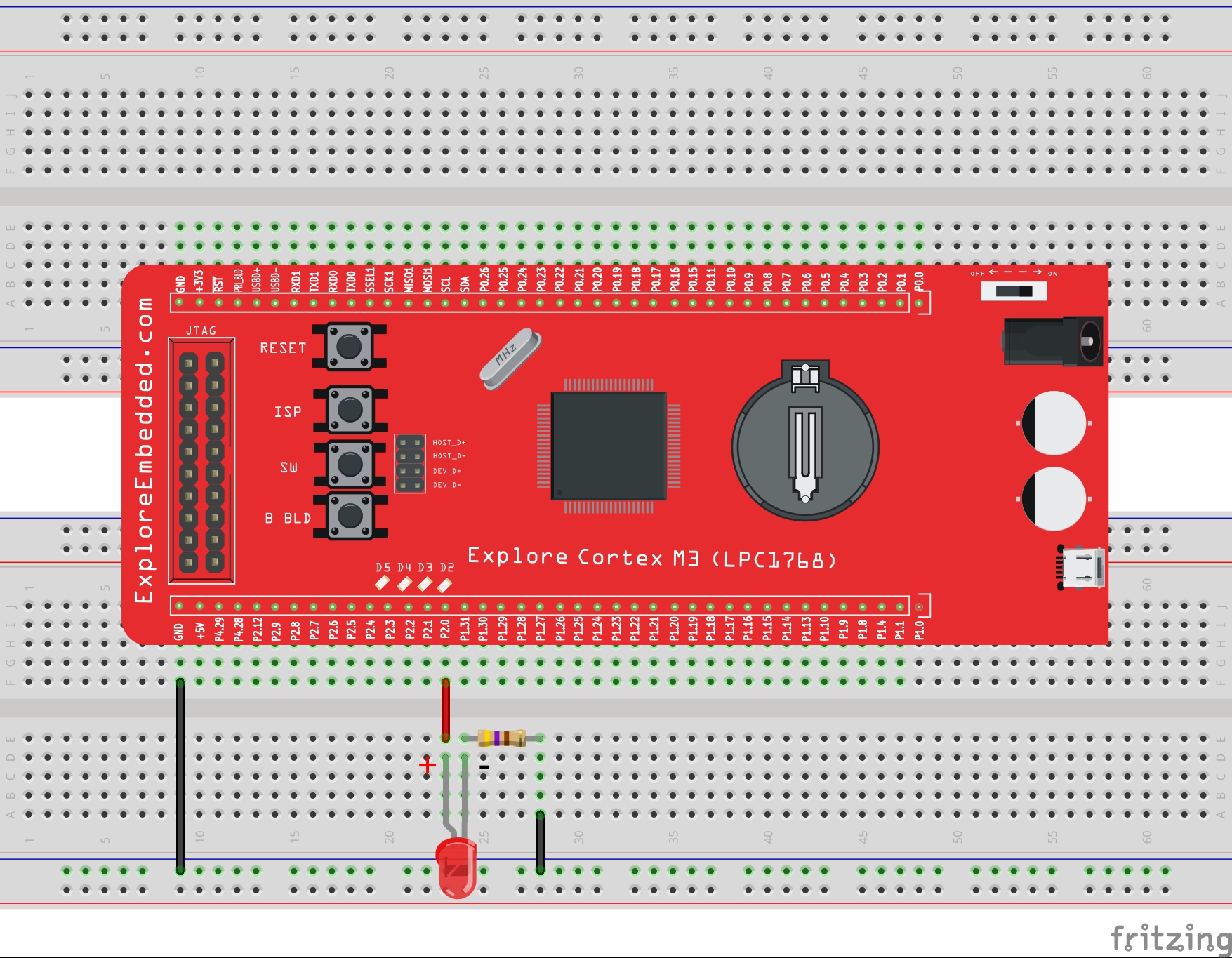
LPC1768: Led Blinking
This is first example on LPC1768 where we start with blinking the LEDs. In this tutorial, we are going to discuss how to configure the LPC1768 ports as GPIO and then send a low/high signal...
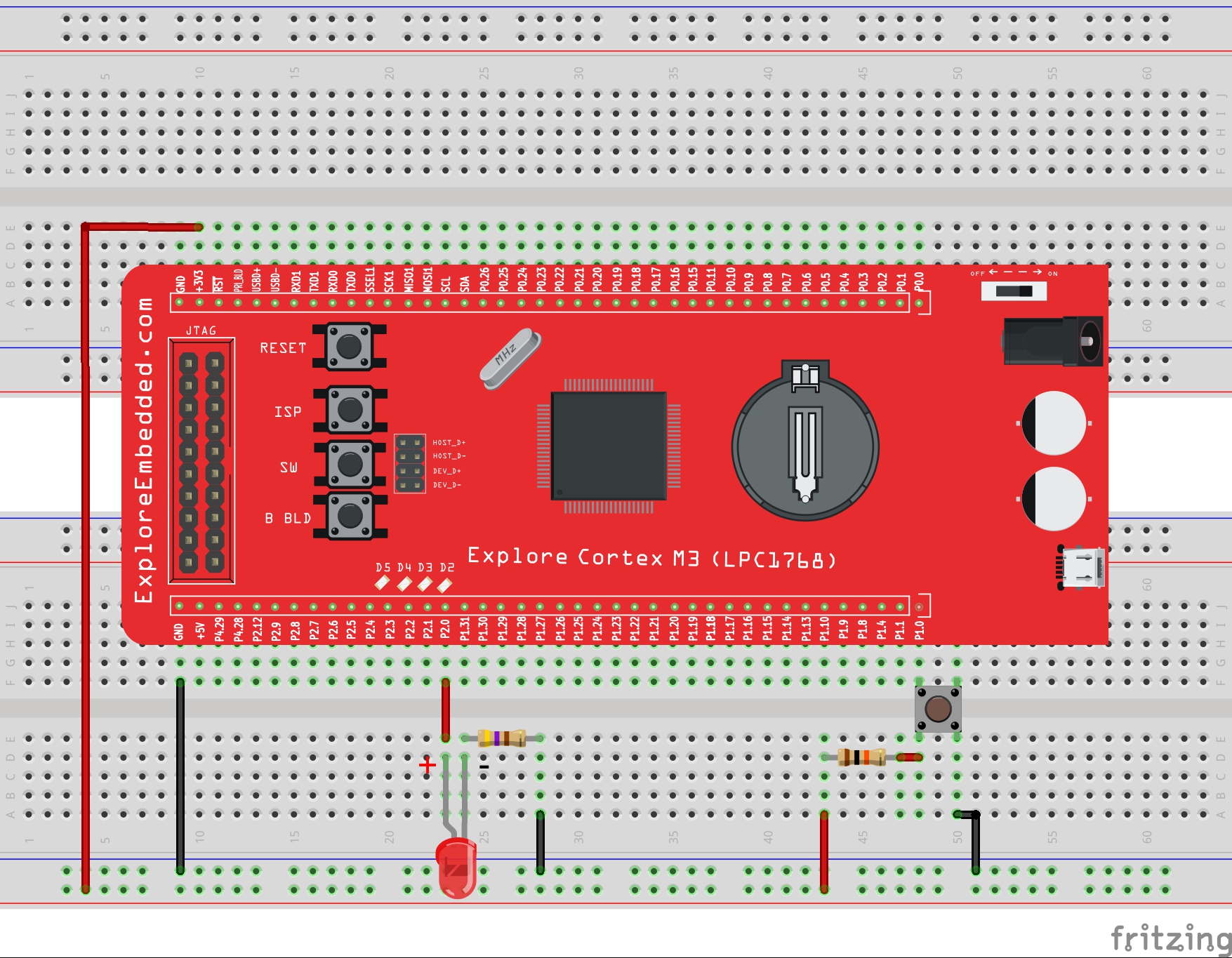
LPC1768: Switch and LED
This is second tutorial on LPC1768 where we are going to read the switches and turn ON/OFF the LEDs accordingly. LPC1768 has its GPIOs divided into five ports PORT0 - PORT4, although many of them...
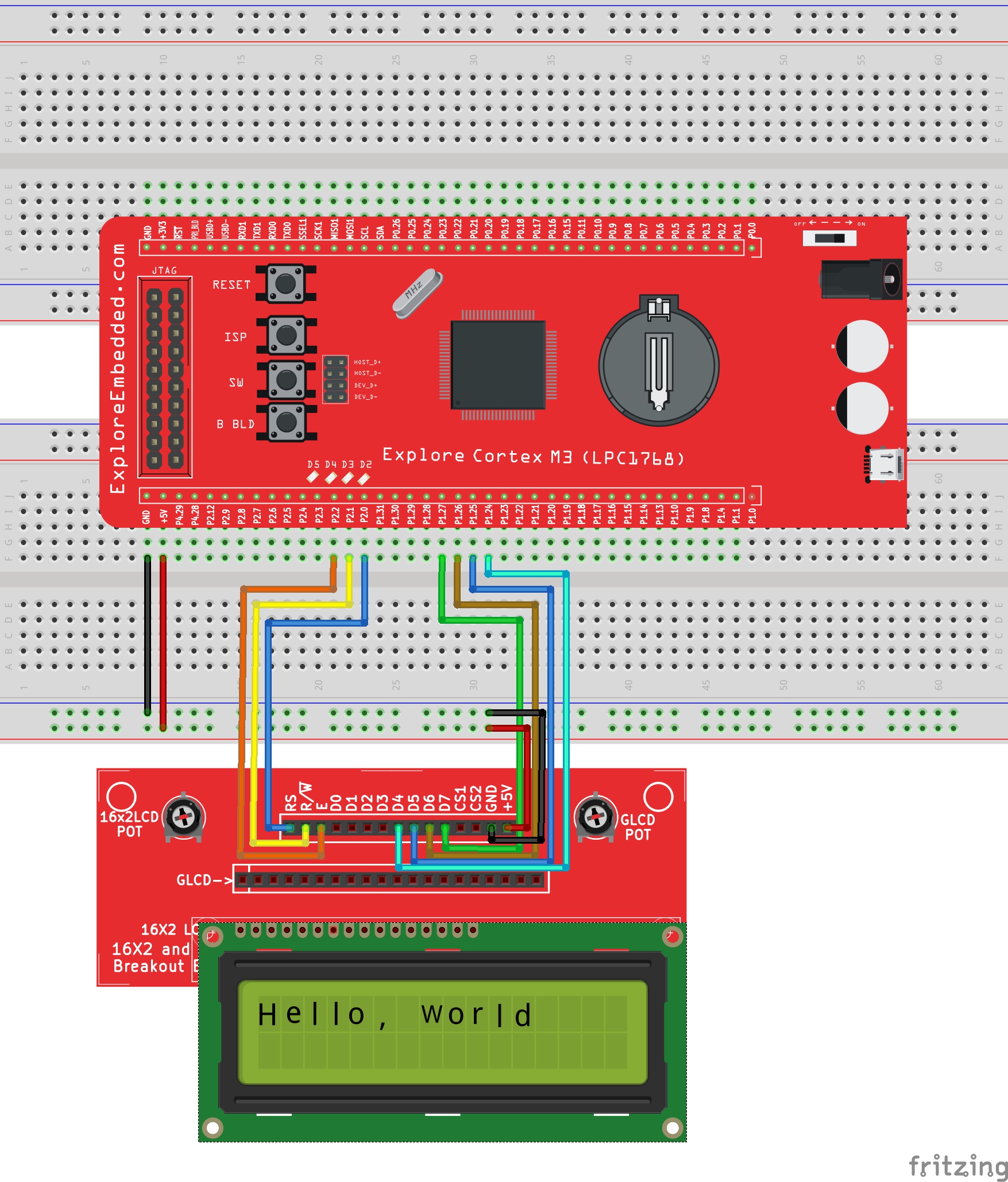
LPC1768: Lcd 4bit
In this tutorial we are going to see how to interface a 2x16 LCD with LPC1768 in 4-bit mode. As per the name the 2x16 has 2 lines with 16 chars on each lines. It supports all the ascii chars and is...

LPC1768: Lcd 8bit
In this tutorial we are going to see how to interface a 2x16 LCD with LPC1768 in 8-bit mode. As per the name the 2x16 has 2 lines with 16 chars on each lines. It supports all the ascii chars and is...