LPC1768: GLCD Interfacing
Contents
[hide]- 1 Objective
- 2 Schematic
- 3 Port Connection
- 4 GLCD Operation
- 5 Code Example
- 5.1 Example 1
- 5.2 Using Explore Embedded Libraries
- 5.3 Explore Embedded Graphics Library
- 5.3.1 GLCD_SetDot ()
- 5.3.2 GLCD_DrawHoriLine()
- 5.3.3 GLCD_DrawVertLine()
- 5.3.4 GLCD_DrawLine()
- 5.3.5 GLCD_DrawRect()
- 5.3.6 GLCD_DrawRoundRect()
- 5.3.7 GLCD_DrawFillRect()
- 5.3.8 GLCD_InvertRect()
- 5.3.9 GLCD_DrawCircle()
- 5.3.10 GLCD_DrawFillCircle()
- 5.3.11 GLCD_DisplayVerticalGraph()
- 5.3.12 GLCD_DisplayHorizontalGraph()
Objective
If you have done with simple 16x2 LCD and still want to do more with display, its time to have fun with Graphics LCD.
We all really enjoy animations kind of things and this is the stuff what we can do with GLCD.
GLCD is very similar to simple 16x2 LCD with additional features. To explore these features and functionality you would like to see our tutorial Graphics LCD Basics : KS0108 based JHD12864E
Schematic
Port Connection
This section shows how to configure the GPIO for interfacing the GLCD.
The below configuration is as per the above schematic. You can connect the GLCD to any of the PORT pins available on your boards and update this section accordingly
/* Configure the data bus and Control bus as per the hardware connection */ | |
#define GlcdDataBusPort LPC_GPIO1->FIOPIN | |
#define GlcdControlBusPort LPC_GPIO2->FIOPIN | |
#define GlcdDataBusDirnReg LPC_GPIO1->FIODIR | |
#define GlcdCtrlBusDirnReg LPC_GPIO2->FIODIR | |
#define GLCD_D0 20 | |
#define GLCD_D1 21 | |
#define GLCD_D2 22 | |
#define GLCD_D3 23 | |
#define GLCD_D4 24 | |
#define GLCD_D5 25 | |
#define GLCD_D6 26 | |
#define GLCD_D7 27 | |
#define GLCD_RS 0 | |
#define GLCD_RW 1 | |
#define GLCD_EN 2 | |
#define GLCD_CS1 3 | |
#define GLCD_CS2 4 |
GLCD Operation
In this section we are going to see how to send the data/cmd to the GLCD along with the timing diagrams. First lets see the timing diagram for sending the data and the command signals(RS,RW,EN), accordingly we write the algorithm and finally the code.
Timing Diagram
The below image shows the timing diagram for sending the data to the GLCD.
As shown in the timing diagram the data is written after sending the RS and RW signals. It is still ok to send the data before these signals.
The only important thing is the data should be available on the databus before generating the High-to-Low pulse on EN pin.
Steps for Sending Command:
- step1: Send the I/P command to GLCD.
- step2: Select the Control Register by making RS low.
- step3: Select Write operation making RW low.
- step4: Send a High-to-Low pulse on Enable PIN with some delay_us.
/* Function to send the command to LCD */ | |
void Glcd_CmdWrite(char cmd) | |
{ | |
sendByte(cmd); //Send the command | |
GlcdControlBusPort &= ~(1<<GLCD_RS); // Send LOW pulse on RS pin for selecting Command register | |
GlcdControlBusPort &= ~(1<<GLCD_RW); // Send LOW pulse on RW pin for Write operation | |
GlcdControlBusPort |= (1<<GLCD_EN); // Generate a High-to-low pulse on EN pin | |
delay(1000); | |
GlcdControlBusPort &= ~(1<<GLCD_EN); | |
delay(10000); | |
} |
Steps for Sending Data:
- step1: Send the character to GLCD.
- step2: Select the Data Register by making RS high.
- step3: Select Write operation making RW low.
- step4: Send a High-to-Low pulse on Enable PIN with some delay_us.
The timings are similar as above only change is that RS is made high for selecting Data register.
/* Function to send the data to GLCD */ | |
void Glcd_DataWrite(char dat) | |
{ | |
sendByte(dat); //Send the data | |
GlcdControlBusPort |= (1<<GLCD_RS); // Send HIGH pulse on RS pin for selecting data register | |
GlcdControlBusPort &= ~(1<<GLCD_RW); // Send LOW pulse on RW pin for Write operation | |
LcdControlBusPort |= (1<<GLCD_EN); // Generate a High-to-low pulse on EN pin | |
delay(1000); | |
GlcdControlBusPort &= ~(1<<GLCD_EN); | |
delay(10000); | |
} |
Code Example
Example 1
Let's start with displaying some text.
#include<lpc17xx.h> | |
/* Configure the data bus and Control bus as per the hardware connection */ | |
#define GlcdDataBusPort LPC_GPIO1->FIOPIN | |
#define GlcdControlBusPort LPC_GPIO2->FIOPIN | |
#define GlcdDataBusDirnReg LPC_GPIO1->FIODIR | |
#define GlcdCtrlBusDirnReg LPC_GPIO2->FIODIR | |
#define GLCD_D0 20 | |
#define GLCD_D1 21 | |
#define GLCD_D2 22 | |
#define GLCD_D3 23 | |
#define GLCD_D4 24 | |
#define GLCD_D5 25 | |
#define GLCD_D6 26 | |
#define GLCD_D7 27 | |
#define GLCD_RS 0 | |
#define GLCD_RW 1 | |
#define GLCD_EN 2 | |
/* Masks for configuring the DataBus and Control Bus direction */ | |
#define PortDataBusConfig ((1<<GLCD_D0)|(1<<GLCD_D1)|(1<<GLCD_D2)|(1<<GLCD_D3)|(1<<GLCD_D4)|(1<<GLCD_D5)|(1<<GLCD_D6)|(1<<GLCD_D7)) | |
#define PortCtrlBusConfig ((1<<GLCD_RS)|(1<<GLCD_RW)|(1<<GLCD_EN)) | |
#define GLCD_dataBusMask ((1<<GLCD_D0)|(1<<GLCD_D1)|(1<<GLCD_D2)|(1<<GLCD_D3)|(1<<GLCD_D4)|(1<<GLCD_D5)|(1<<GLCD_D6)|(1<<GLCD_D7)) | |
/* local function to generate some delay */ | |
void delay(int cnt) | |
{ | |
int i; | |
for(i=0;i<cnt;i++); | |
} | |
/* Function send a complete byte on the Data bus */ | |
void sendByte(char byte) | |
{ | |
GlcdDataBusPort&=~(GLCD_dataBusMask); // Clear previous data | |
GlcdDataBusPort|= (((byte >>0x00) & 0x01) << GLCD_D0); | |
GlcdDataBusPort|= (((byte >>0x01) & 0x01) << GLCD_D1); | |
GlcdDataBusPort|= (((byte >>0x02) & 0x01) << GLCD_D2); | |
GlcdDataBusPort|= (((byte >>0x03) & 0x01) << GLCD_D3); | |
GlcdDataBusPort|= (((byte >>0x04) & 0x01) << GLCD_D4); | |
GlcdDataBusPort|= (((byte >>0x05) & 0x01) << GLCD_D5); | |
GlcdDataBusPort|= (((byte >>0x06) & 0x01) << GLCD_D6); | |
GlcdDataBusPort|= (((byte >>0x07) & 0x01) << GLCD_D7); | |
} | |
/* Function to send the command to Glcd */ | |
void Glcd_CmdWrite(char cmd) | |
{ | |
sendByte(cmd); //Send the Command | |
GlcdControlBusPort &= ~(1<<GLCD_RS); // Send LOW pulse on RS pin for selecting Command register | |
GlcdControlBusPort &= ~(1<<GLCD_RW); // Send LOW pulse on RW pin for Write operation | |
GlcdControlBusPort |= (1<<GLCD_EN); // Generate a High-to-low pulse on EN pin | |
delay(1000); | |
GlcdControlBusPort &= ~(1<<GLCD_EN); | |
delay(10000); | |
} | |
/* Function to send the data to Glcd */ | |
void Glcd_DataWrite(char dat) | |
{ | |
sendByte(dat); //Send the data | |
GlcdControlBusPort |= (1<<GLCD_RS); // Send HIGH pulse on RS pin for selecting data register | |
GlcdControlBusPort &= ~(1<<GLCD_RW); // Send LOW pulse on RW pin for Write operation | |
GlcdControlBusPort |= (1<<GLCD_EN); // Generate a High-to-low pulse on EN pin | |
delay(1000); | |
GlcdControlBusPort &= ~(1<<GLCD_EN); | |
delay(10000); | |
} | |
void Glcd_SelectPage0() | |
{ | |
GlcdControlBusPort |= (1<<GLCD_CS1); | |
GlcdControlBusPort &= ~(1<<GLCD_CS2); | |
} | |
void Glcd_SelectPage1() | |
{ | |
GlcdControlBusPort |= (1<<GLCD_CS2); | |
GlcdControlBusPort &= ~(1<<GLCD_CS1); | |
} | |
void main() | |
{ | |
char i,a[]={"Good morning!"}; | |
SystemInit(); //Clock and PLL configuration | |
GlcdDataBusDirnReg = PortDataBusConfig; // Configure all the Glcd pins as output | |
GlcdCtrlBusDirnReg = PortCtrlBusConfig; | |
/* Select the Page0/Page1 and turn ON the GLCD */ | |
Glcd_SelectPage0(); | |
Glcd_CmdWrite(0x3f); | |
Glcd_SelectPage1(); | |
Glcd_CmdWrite(0x3f); | |
DELAY_ms(10); | |
/* Select the Page0/Page1 and Enable the GLCD */ | |
Glcd_SelectPage0(); | |
Glcd_CmdWrite(0xc0); | |
Glcd_SelectPage1(); | |
Glcd_CmdWrite(0xc0); | |
Glcd_SelectPage0(); | |
Glcd_CmdWrite(0xB8); // Move the cursor to first line | |
Glcd_CmdWrite(0x40); // Move the cursor to beginning of line | |
Glcd_DataWrite('H'); | |
Glcd_DataWrite('e'); | |
Glcd_DataWrite('l'); | |
Glcd_DataWrite('l'); | |
Glcd_DataWrite('o'); | |
Glcd_DataWrite(' '); | |
Glcd_SelectPage1(); | |
Glcd_DataWrite('w'); | |
Glcd_DataWrite('o'); | |
Glcd_DataWrite('r'); | |
Glcd_DataWrite('l'); | |
Glcd_DataWrite('d'); | |
Glcd_SelectPage0(); | |
Glcd_CmdWrite(0xB9); // Move the cursor to second line | |
Glcd_CmdWrite(0x40); // Move the cursor to beginning of line | |
for(i=0;a[i]!=0;i++) | |
{ | |
Glcd_DataWrite(a[i]); | |
} | |
while(1); | |
} |
Using Explore Embedded Libraries
In the above example we just discussed how to interface Graphics Lcd.
Once you know the working of GLCD, you can directly use the ExploreEmbedded libraries to play around with your GLCD.
For that you need to include the glcd.c/glcd.h and the associated files(delay/stdutils).
The below sample code shows how to use the already available GLCD functions.
Refer this link for more info on GLCD libraries.
#include<lpc17xx.h> | |
#include "glcd.h" //User defined GLCD library which contains the lcd routines | |
#include "delay.h" //User defined library which contains the delay routines | |
void main() | |
{ | |
SystemInit(); //Clock and PLL configuration | |
/* Setup/Map the controller pins for LCD operation | |
CS1 CS2 RS RW EN D0 D1 D2 D3 D4 D5 D6 D7 */ | |
GLCD_SetUp(P2_0,P2_1,P2_2,P2_3,P2_4,P1_20,P1_21,P1_22,P1_23,P1_24,P1_25,P1_26,P1_27); | |
/* Initialize GLCD before use */ | |
GLCD_Init(); | |
while(1) | |
{ | |
/*scroll the message on line1 */ | |
GLCD_Clear(); | |
GLCD_ScrollMessage(1," Program to demonstrate the ExploreEmbedded Lib Usage"); | |
GLCD_Clear(); | |
GLCD_Printf("Hello, World \nGood Morning"); | |
DELAY_sec(3); | |
GLCD_Clear(); | |
GLCD_Printf("DecNum:%4d",1234); | |
GLCD_Printf("\nHexNum:%4x",0xABCD); | |
DELAY_sec(3); | |
GLCD_Clear(); | |
GLCD_Printf("16-bit Bin:1234"); | |
GLCD_GoToNextLine(); | |
GLCD_Printf("%16b",0x1234); | |
DELAY_sec(3); | |
} | |
} |
Explore Embedded Graphics Library
GLCD_SetDot ()
{{#Widget:LibTable}}Defination | void GLCD_SetDot ( uint8_t var_x_u8, uint8_t var_y_u8, uint8_t var_color_u8 ) | ||||||||||||||||
Input Arguments |
| ||||||||||||||||
Return Value | none | ||||||||||||||||
Description | This function is used to draw a dot | ||||||||||||||||
Usage |
This file contains hidden or bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
| ||||||||||||||||
Output | ![]() |
GLCD_DrawHoriLine()
{{#Widget:LibTable}}Defination | void GLCD_DrawHoriLine(uint8_t var_x_u8, uint8_t var_y_u8, uint8_t var_length_u8,uint8_t var_color_u8) | ||||||||||||||||||||||
Input Arguments |
| ||||||||||||||||||||||
Return Value | none | ||||||||||||||||||||||
Description | This function is used to draw horizontial line. | ||||||||||||||||||||||
Usage |
This file contains hidden or bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
| ||||||||||||||||||||||
Output | ![]() |
GLCD_DrawVertLine()
{{#Widget:LibTable}}Defination | void GLCD_DrawVertLine(uint8_t var_x_u8, uint8_t var_y_u8, uint8_t var_length_u8,uint8_t var_color_u8) | ||||||||||||||||||||||
Input Arguments |
| ||||||||||||||||||||||
Return Value | none | ||||||||||||||||||||||
Description | This function is used to draw vertical line. | ||||||||||||||||||||||
Usage |
This file contains hidden or bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
| ||||||||||||||||||||||
Output | ![]() |
GLCD_DrawLine()
{{#Widget:LibTable}}Defination | void GLCD_DrawLine ( uint8_t var_x1_u8, uint8_t var_y1_u8, uint8_t var_x2_u8, uint8_t var_y2_u8, uint8_t var_color_u8) | ||||||||||
Input Arguments |
| ||||||||||
Return Value | none | ||||||||||
Description | This function is used to draw line. | ||||||||||
Usage |
This file contains hidden or bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
| ||||||||||
Output | ![]() |
GLCD_DrawRect()
{{#Widget:LibTable}}Defination | void GLCD_DrawRect(uint8_t var_x_u8, uint8_t var_y_u8, uint8_t var_width_u8, uint8_t var_height_u8, uint8_t var_color_u8) | ||||||||
Input Arguments |
| ||||||||
Return Value | none | ||||||||
Description | This function is used to draw rectangle. | ||||||||
Usage |
This file contains hidden or bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
| ||||||||
Output | ![]() |
GLCD_DrawRoundRect()
{{#Widget:LibTable}}Defination | void GLCD_DrawRoundRect(uint8_t var_x_u8, uint8_t var_y_u8, uint8_t var_width_u8, uint8_t var_height_u8, var_radius_u8, uint8_t var_color_u8) | ||||||
Input Arguments |
| ||||||
Return Value | none | ||||||
Description | This function is used to draw round rectangle. | ||||||
Usage |
This file contains hidden or bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
| ||||||
Output | ![]() |
GLCD_DrawFillRect()
{{#Widget:LibTable}}GLCD_InvertRect()
{{#Widget:LibTable}}GLCD_DrawCircle()
{{#Widget:LibTable}}GLCD_DrawFillCircle()
{{#Widget:LibTable}}GLCD_DisplayVerticalGraph()
{{#Widget:LibTable}}GLCD_DisplayHorizontalGraph()
{{#Widget:LibTable}}
Lots of things are there which you would like to do with GLCD and we will cover it in the future tutorials. For now, you don't forget to comment.
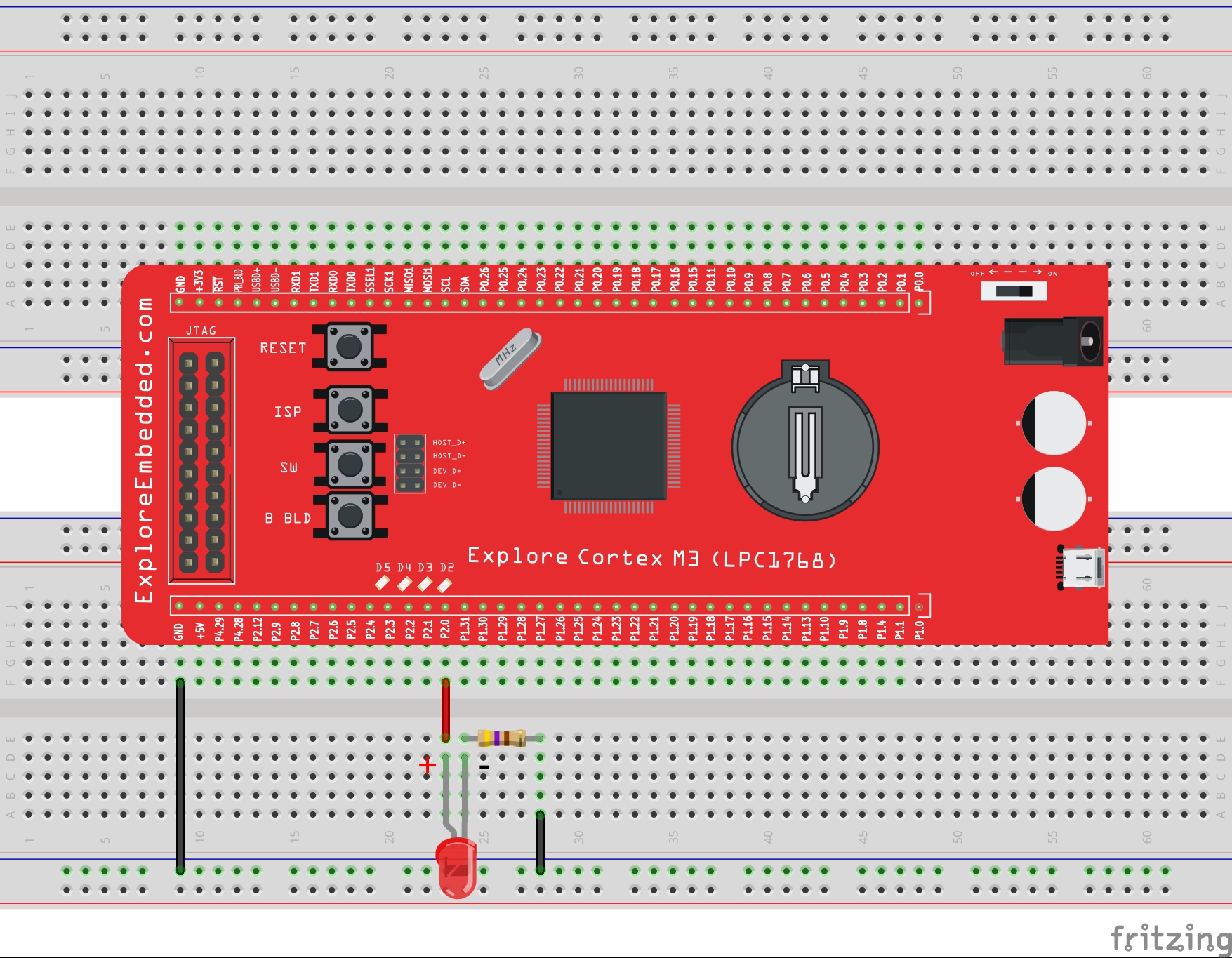
LPC1768: Led Blinking
This is first example on LPC1768 where we start with blinking the LEDs. In this tutorial, we are going to discuss how to configure the LPC1768 ports as GPIO and then send a low/high signal...
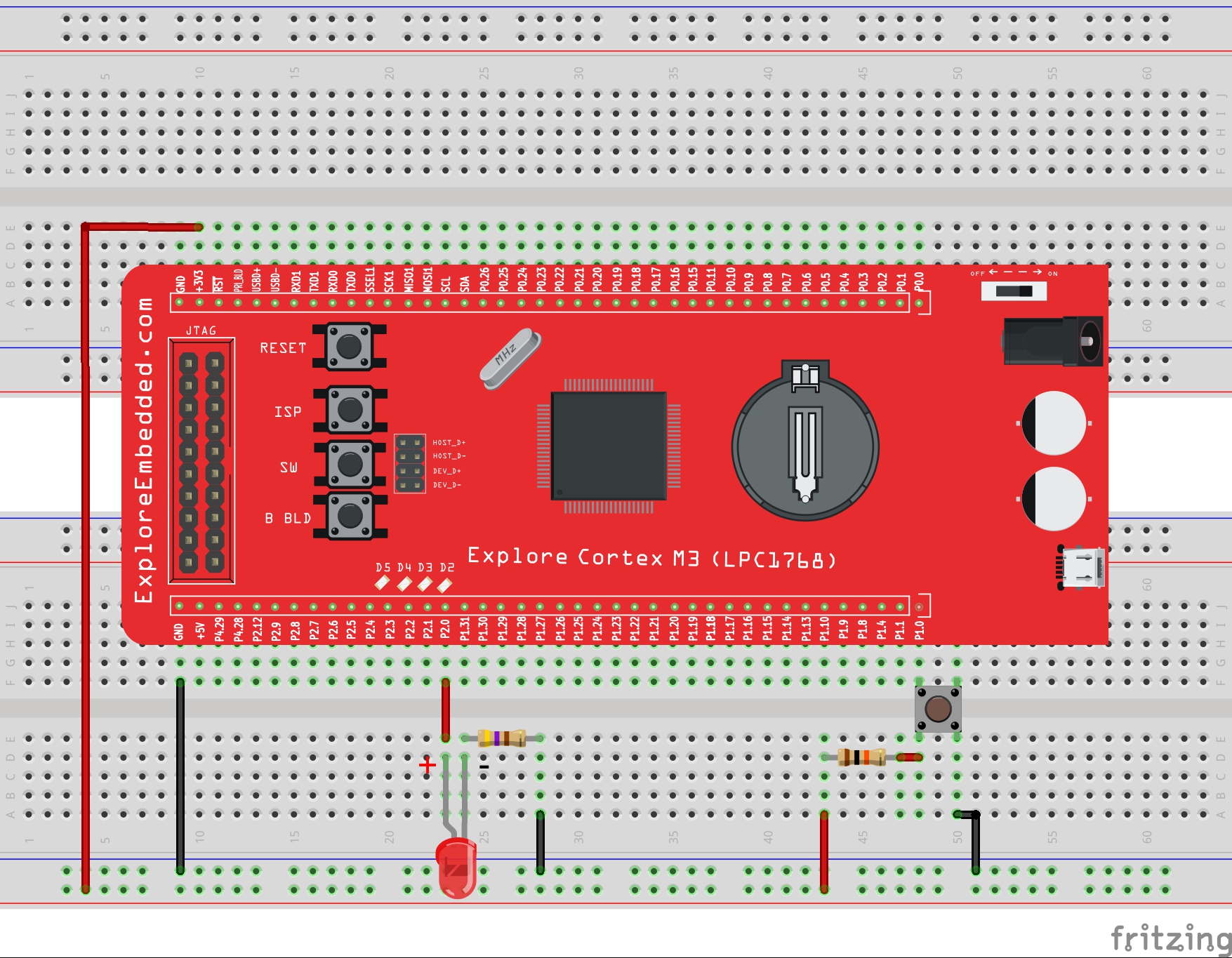
LPC1768: Switch and LED
This is second tutorial on LPC1768 where we are going to read the switches and turn ON/OFF the LEDs accordingly. LPC1768 has its GPIOs divided into five ports PORT0 - PORT4, although many of them...
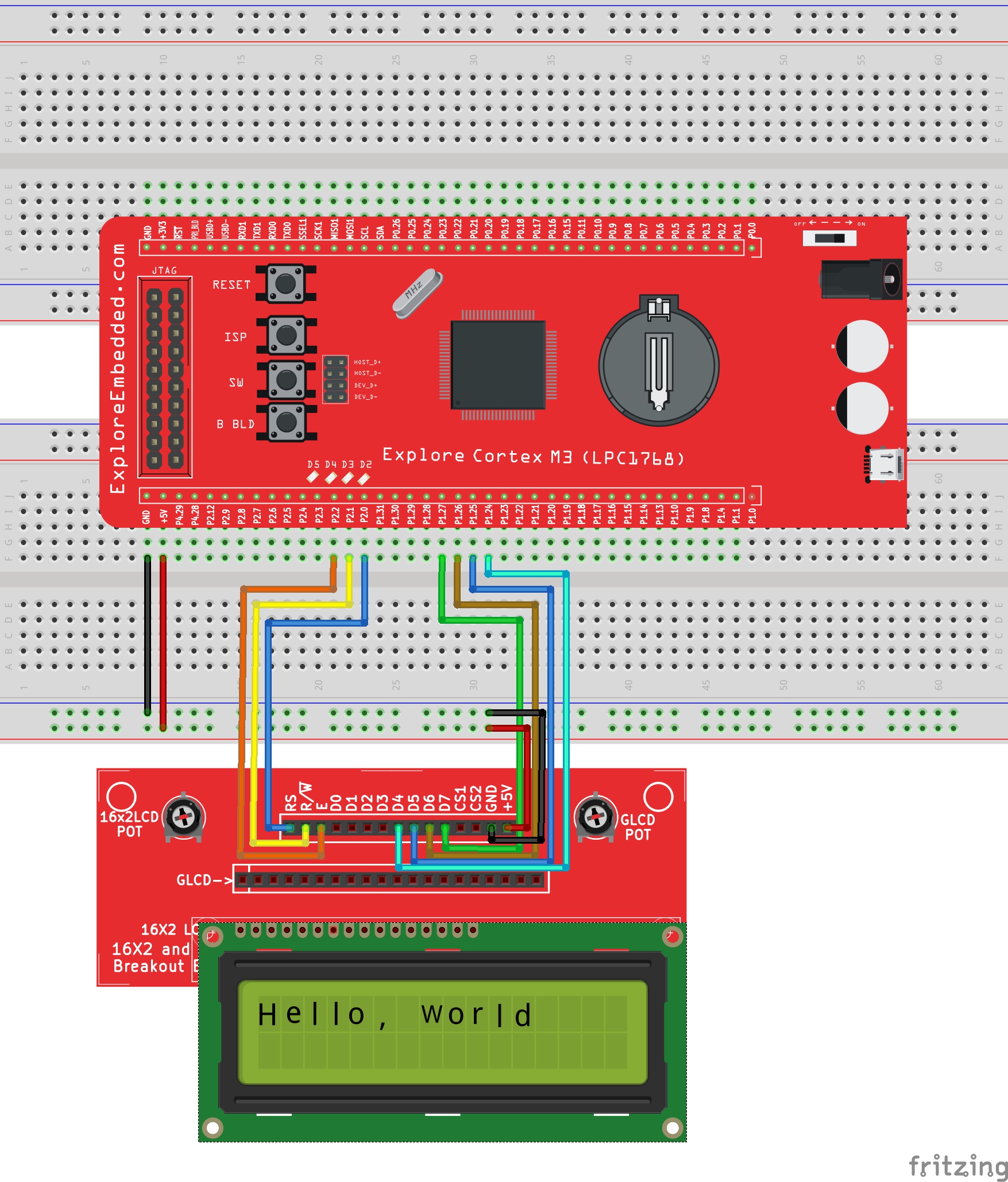
LPC1768: Lcd 4bit
In this tutorial we are going to see how to interface a 2x16 LCD with LPC1768 in 4-bit mode. As per the name the 2x16 has 2 lines with 16 chars on each lines. It supports all the ascii chars and is...

LPC1768: Lcd 8bit
In this tutorial we are going to see how to interface a 2x16 LCD with LPC1768 in 8-bit mode. As per the name the 2x16 has 2 lines with 16 chars on each lines. It supports all the ascii chars and is...